์ค๋ ํ ๊ณต๋ถ ๐ง
- ์๊ณ ๋ฆฌ์ฆ ๋ฌธ์ (Java, SQL) 1๋ฌธ์ ํ์ด & ๋ธ๋ก๊ทธ ์ ๋ฆฌ
- Spring ์๋ จ ๊ฐ์ ๋ฃ๊ธฐ (3์ฃผ์ฐจ ์ค์ต ํํธ)
- Spring ์๋ จ ๊ฐ์ ๋ด์ฉ ๋ณต์ต
- TIL ๋ธ๋ก๊ทธ ์์ฑ
์ค๋ ์ป์ ๋ด์ฉ ์ ๋ฆฌ โ๏ธ
์ค์ต ํํธ ๋ณต์ตํ๋ฉด์ ๋ด์ฉ๋ค ์์๋๋ก ์ ๋ฆฌํด์ผ๊ฒ ๋ค.
1. JPA ๊ด๋ จ ์ค์ ํ๊ธฐ
DataSource ์ค์
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/board
spring.datasource.username=๊ณ์
spring.datasource.password=๋น๋ฐ๋ฒํธ
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Hibernate ์ค์
application.properties
spring.jpa.hibernate.ddl-auto=create
spring.jpa.properties.hibernate.show_sql=true
spring.jpa.properties.hibernate.format_sql=true
spring.jpa.properties.hibernate.use_sql_comments=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
2. Entity ์ค๊ณ
BaseEntity ์ค๊ณ ๋ฐ ์ ์ฉํ๊ธฐ
1. BaseEntity ํด๋์ค
ํ๋๋ช | ํ์ | ์ค๋ช |
createdAt | LocalDateTime | ์์ฑ ์๊ฐ |
updatedAt | LocalDateTime | ์์ ์๊ฐ |
2. BaseEntity Entity ๊ตฌํ
@Getter
@MappedSuperclass // ํด๋น ์ด๋
ธํ
์ด์
์ด ์ ์ธ๋ ํด๋์ค๋ฅผ ์์๋ฐ๋ Entity์ ๊ณตํต ๋งคํ ์ ๋ณด๋ฅผ ์ ๊ณตํ๋ค.
@EntityListeners(AuditingEntityListener.class) // JPA Entity์ ์๋ช
์ฃผ๊ธฐ ์ด๋ฒคํธ๋ฅผ ๊ฐ์งํ๊ณ , ํด๋น ์ด๋ฒคํธ์ ๋ฐ๋ผ ํน์ ๋ก์ง์ ์คํํ ์ ์๋๋ก ์ค์ ํ๋ Annotation
public abstract class BaseEntity {
@CreatedDate
@Column(updatable = false)
// @Temporal(TemporalType.TIMESTAMP) // ์๋ต ๊ฐ๋ฅํ๋ค.
private LocalDateTime createdAt;
@LastModifiedDate
private LocalDateTime updatedAt;
}
3. @EnableJpaAuditing ์ ์ฉ
@EnableJpaAuditing
@SpringBootApplication
public class BoardApplication {
public static void main(String[] args) {
SpringApplication.run(JpaBoardApplication.class, args);
}
}
ํ์(Member) ์ค๊ณํ๊ธฐ
1. Member ํด๋์ค
ํ๋๋ช | ํ์ | ์ค๋ช |
id | Long | ์๋ณ์ |
username | String | ๋ก๊ทธ์ธ์ ์ฌ์ฉํ ์์ด๋ |
password | String | ๋ก๊ทธ์ธ์ ์ฌ์ฉํ ๋น๋ฐ๋ฒํธ |
age | Integer | ๋์ด |
createdAt | LocalDateTime | ์์ฑ ์๊ฐ |
updatedAt | LocalDateTime | ์์ ์๊ฐ |
2. Member Entity ๊ตฌํ
@Entity
@Table(name = "member")
public class Member extends BaseEntity{
// ์์ฑ
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY) // JPA์์ ๊ธฐ๋ณธ ํค(PK)๊ฐ์ ์๋์ผ๋ก ์์ฑํ ๋ ์ฌ์ฉํ๋ ๋ฐฉ๋ฒ ์ค ํ๋์ด๋ค. GenerationType.IDENTITY๋ MySQL ๋ฐ์ดํฐ๋ฒ ์ด์ค๋ฅผ ์ฌ์ฉํด์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ์๋ ์ฆ๊ฐ ๊ธฐ๋ฅ์ ์ฌ์ฉํด ๊ธฐ๋ณธ ํค๋ฅผ ์์ฑํ๋ค.
private Long id; // ๊ณ ์ ์๋ณ์ (์๋์ผ๋ก ์์ฑ)
@Column(nullable = false, unique = true) // nullable์ ๊ธฐ๋ณธ๊ฐ์ true์ด๋ค.
private String username; // ๋ก๊ทธ์ธํ ๋ ์ฌ์ฉํ๋ ์์ด๋
@Column(nullable = false)
private String password; // ๋ก๊ทธ์ธํ ๋ ์ฌ์ฉํ๋ ๋น๋ฐ๋ฒํธ
private Integer age; // ๋์ด
// ์์ฑ์
// ๊ธฐ๋ฅ
}
3. Application ์คํ ๋ฐ ๊ฒฐ๊ณผ ํ์ธ
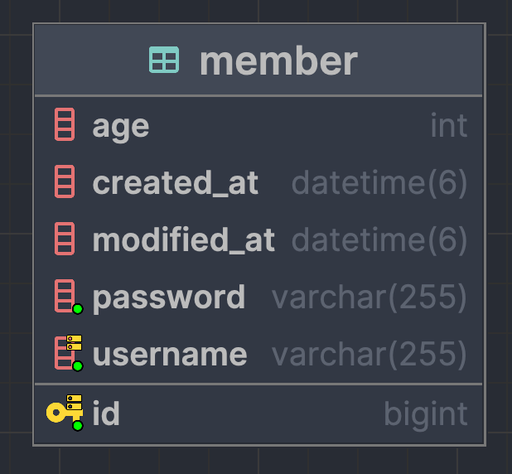
๊ฒ์๊ธ(Board) ์ค๊ณํ๊ธฐ
1. Board ํด๋์ค
ํ๋๋ช | ํ์ | ์ค๋ช |
id | Long | ์๋ณ์ |
title | String | ์ ๋ชฉ |
contents | String | ๋ด์ฉ |
createdAt | LocalDateTime | ์์ฑ ์๊ฐ |
updatedAt | LocalDateTime | ์์ ์๊ฐ |
2. Board Entity ๊ตฌํ
@Entity
@Table(name = "board")
public class Board extends BaseEntity{
// ์์ฑ
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(columnDefinition = "longtext")
private String contents;
// ์์ฑ์
// ๊ธฐ๋ฅ
}
3. Application ์คํ ๋ฐ ๊ฒฐ๊ณผ ํ์ธ

3. ์ฐ๊ด๊ด๊ณ ์ค์
์ฐ๊ด๊ด๊ณ ์ค์ ํ๊ธฐ
- ํ ๋ช ์ ํ์์ ์ฌ๋ฌ ๊ฐ์ ๊ฒ์๊ธ์ ์์ฑํ ์ ์๋ค. (N:1 ๋จ๋ฐฉํฅ)
1. Board Entity
@Entity
@Table(name = "board")
public class Board extends BaseEntity{
// ์์ฑ
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(columnDefinition = "longtext")
private String contents;
@ManyToOne
@JoinColumn(name = "member_id")
private Member member;
// ์์ฑ์
// ๊ธฐ๋ฅ
}
2. Application ์คํ ๋ฐ ๊ฒฐ๊ณผ ํ์ธ
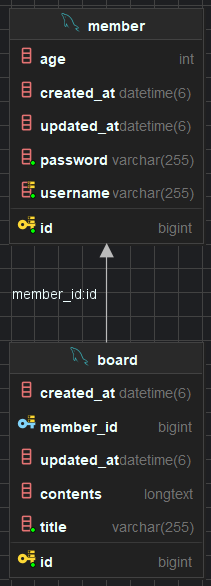
4. ํ์ ์์ฑ ๊ธฐ๋ฅ
Member ์์ฑ API ๊ตฌํ
SignUpRequestDto
@Getter
public class SignUpReqeustDto {
// ์์ฑ
private final String username;
private final String password;
private final Integer age;
// ์์ฑ์
public SignUpReqeustDto(String username, String password, Integer age) {
this.username = username;
this.password = password;
this.age = age;
}
// ๊ธฐ๋ฅ
}
SignUpResponseDto
@Getter
public class SignUpResponseDto {
// ์์ฑ
private final Long id;
private final String username;
private final Integer age;
// ์์ฑ์
public SignUpResponseDto(Long id, String username, Integer age) {
this.id = id;
this.username = username;
this.age = age;
}
// ๊ธฐ๋ฅ
}
Member
@Getter
@Entity
@Table(name = "member")
public class Member extends BaseEntity{
// ์์ฑ
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY) // JPA์์ ๊ธฐ๋ณธ ํค(PK)๊ฐ์ ์๋์ผ๋ก ์์ฑํ ๋ ์ฌ์ฉํ๋ ๋ฐฉ๋ฒ ์ค ํ๋์ด๋ค. GenerationType.IDENTITY๋ MySQL ๋ฐ์ดํฐ๋ฒ ์ด์ค๋ฅผ ์ฌ์ฉํด์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ์๋ ์ฆ๊ฐ ๊ธฐ๋ฅ์ ์ฌ์ฉํด ๊ธฐ๋ณธ ํค๋ฅผ ์์ฑํ๋ค.
private Long id; // ๊ณ ์ ์๋ณ์ (์๋์ผ๋ก ์์ฑ)
@Column(nullable = false, unique = true) // nullable์ ๊ธฐ๋ณธ๊ฐ์ true์ด๋ค.
private String username; // ๋ก๊ทธ์ธํ ๋ ์ฌ์ฉํ๋ ์์ด๋
@Column(nullable = false)
private String password; // ๋ก๊ทธ์ธํ ๋ ์ฌ์ฉํ๋ ๋น๋ฐ๋ฒํธ
private Integer age; // ๋์ด
// ์์ฑ์
public Member() {}
public Member(String username, String password, Integer age) {
this.username = username;
this.password = password;
this.age = age;
}
// ๊ธฐ๋ฅ
}
MemberController
@RestController
@RequestMapping("/members")
@RequiredArgsConstructor
public class MemberController {
// ์์ฑ
private final MemberService memberService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@PostMapping("/signup")
public ResponseEntity<SignUpResponseDto> signUp(@RequestBody SignUpReqeustDto reqeustDto) {
SignUpResponseDto signUpResponseDto = memberService.signUp(reqeustDto.getUsername(), reqeustDto.getPassword(), reqeustDto.getAge());
return new ResponseEntity<>(signUpResponseDto, HttpStatus.CREATED);
}
}
MemberService
@Service
@RequiredArgsConstructor
public class MemberService {
// ์์ฑ
private final MemberRepository memberRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public SignUpResponseDto signUp(String username, String password, Integer age) {
Member member = new Member(username, password, age);
Member savedMember = memberRepository.save(member);
return new SignUpResponseDto(savedMember.getId(), savedMember.getUsername(), savedMember.getAge());
}
}
- ํ์ฅํ ๊ฐ๋ฅ์ฑ์ด ์๋ค๋ฉด Interface๊ฐ ์๋ ๊ตฌํ์ฒด๋ฅผ ์์กดํด๋ ๋๋ค.
MemberRepository
public interface MemberRepository extends JpaRepository<Member, Long> {
// ์์ฑ
// ์์ฑ์
// ๊ธฐ๋ฅ
}
5. ํน์ ํ์ ์กฐํ ๊ธฐ๋ฅ
ํน์ Member ์กฐํ API ๊ตฌํ
MemberResponseDto
@Getter
public class MemberResponseDto {
// ์์ฑ
private final String username;
private final Integer age;
// ์์ฑ์
public MemberResponseDto(String username, Integer age) {
this.username = username;
this.age = age;
}
// ๊ธฐ๋ฅ
}
MemberController
@RestController
@RequestMapping("/members")
@RequiredArgsConstructor
public class MemberController {
// ์์ฑ
private final MemberService memberService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@GetMapping("/{id}")
public ResponseEntity<MemberResponseDto> findById(@PathVariable Long id) {
MemberResponseDto memberResponseDto = memberService.findById(id);
return new ResponseEntity<>(memberResponseDto, HttpStatus.OK);
}
}
MemberService
@Service
@RequiredArgsConstructor
public class MemberService {
// ์์ฑ
private final MemberRepository memberRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public MemberResponseDto findById(Long id) {
Optional<Member> optionalMember = memberRepository.findById(id);
if (optionalMember.isEmpty()) {
throw new ResponseStatusException(HttpStatus.NOT_FOUND, "Does not exist id = " + id);
}
Member findMember = optionalMember.get();
return new MemberResponseDto(findMember.getUsername(), findMember.getAge());
}
}
6. ๋น๋ฐ๋ฒํธ ์์ ๊ธฐ๋ฅ
๋น๋ฐ๋ฒํธ ์์ API ๊ตฌํ
UpdatePasswordRequestDto
@Getter
public class UpdatePasswordRequestDto {
// ์์ฑ
private final String oldPassword;
private final String newPassword;
// ์์ฑ์
public UpdatePasswordRequestDto(String oldPassword, String newPassword) {
this.oldPassword = oldPassword;
this.newPassword = newPassword;
}
// ๊ธฐ๋ฅ
}
MemberController
@RestController
@RequestMapping("/members")
@RequiredArgsConstructor
public class MemberController {
// ์์ฑ
private final MemberService memberService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@PatchMapping("/{id}")
public ResponseEntity<Void> updatePassword(
@PathVariable Long id,
@RequestBody UpdatePasswordRequestDto requestDto
) {
memberService.updatePassword(id, requestDto.getOldPassword(), requestDto.getNewPassword());
return new ResponseEntity<>(HttpStatus.OK);
}
}
MemberService
@Service
@RequiredArgsConstructor
public class MemberService {
// ์์ฑ
private final MemberRepository memberRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
@Transactional
public void updatePassword(long id, String oldPassword, String newPassword) {
Member findMember = memberRepository.findByIdOrElseThrow(id);
if (!findMember.getPassword().equals(oldPassword)) {
throw new ResponseStatusException(HttpStatus.UNAUTHORIZED, "๋น๋ฐ๋ฒํธ๊ฐ ์ผ์นํ์ง ์์ต๋๋ค.");
}
findMember.updatePassword(newPassword);
}
}
Member
public void updatePassword(String password) {
this.password = password;
}
MemberRepository
public interface MemberRepository extends JpaRepository<Member, Long> {
// ์์ฑ
// ์์ฑ์
// ๊ธฐ๋ฅ
default Member findByIdOrElseThrow(Long id) {
return findById(id).orElseThrow(() -> new ResponseStatusException(HttpStatus.NOT_FOUND, "Does not exist id = " + id));
}
}
7. ๊ฒ์๊ธ ์์ฑ ๊ธฐ๋ฅ
๊ฒ์๊ธ ์์ฑ API ๊ตฌํ
CreateBoardRequestDto
@Getter
public class CreateBoardReqeustDto {
// ์์ฑ
private final String title;
private final String contents;
private final String username;
// ์์ฑ์
public CreateBoardReqeustDto(String title, String contents, String username) {
this.title = title;
this.contents = contents;
this.username = username;
}
// ๊ธฐ๋ฅ
}
BoardResponseDto
@Getter
public class BoardResponseDto {
// ์์ฑ
private final Long id;
private final String title;
private final String contents;
// ์์ฑ์
public BoardResponseDto(Long id, String title, String contents) {
this.id = id;
this.title = title;
this.contents = contents;
}
// ๊ธฐ๋ฅ
}
Board
@Getter
@Entity
@Table(name = "board")
public class Board extends BaseEntity{
// ์์ฑ
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(columnDefinition = "longtext")
private String contents;
@ManyToOne
@JoinColumn(name = "member_id")
private Member member;
// ์์ฑ์
public Board() {}
public Board(String title, String contents) {
this.title = title;
this.contents = contents;
}
// ๊ธฐ๋ฅ
public void setMember(Member member) {
this.member = member;
}
}
BoardController
@RestController
@RequestMapping("/boards")
@RequiredArgsConstructor
public class BoardController {
// ์์ฑ
private final BoardService boardService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@PostMapping
public ResponseEntity<BoardResponseDto> save(@RequestBody CreateBoardReqeustDto reqeustDto) {
BoardResponseDto boardResponseDto = boardService.save(reqeustDto.getTitle(), reqeustDto.getContents(), reqeustDto.getUsername());
return new ResponseEntity<>(boardResponseDto, HttpStatus.CREATED);
}
}
BoardService
@Service
@RequiredArgsConstructor
public class BoardService {
// ์์ฑ
private final MemberRepository memberRepository;
private final BoardRepository boardRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public BoardResponseDto save(String title, String contents, String username) {
Member findMember = memberRepository.findMemberByUsernameOrElseThrow(username);
Board board = new Board(title, contents);
board.setMember(findMember);
Board savedBoard = boardRepository.save(board);
return new BoardResponseDto(savedBoard.getId(), savedBoard.getTitle(), savedBoard.getContents());
}
}
MemberRepository
public interface MemberRepository extends JpaRepository<Member, Long> {
// ์์ฑ
// ์์ฑ์
// ๊ธฐ๋ฅ
Optional<Member> findMemberByUsername(String username);
default Member findMemberByUsernameOrElseThrow(String username) {
return findMemberByUsername(username).orElseThrow(() -> new ResponseStatusException(HttpStatus.NOT_FOUND, "Does not exist username = " + username));
}
}
BoardRepository
public interface BoardRepository extends JpaRepository<Board, Long> {
}
8. ๊ฒ์๊ธ ์ ์ฒด ์กฐํ ๊ธฐ๋ฅ
๊ฒ์๊ธ ์ ์ฒด ์กฐํ API ๊ตฌํ
BoardResponseDto
@Getter
public class BoardResponseDto {
// ์์ฑ
private final Long id;
private final String title;
private final String contents;
// ์์ฑ์
public BoardResponseDto(Long id, String title, String contents) {
this.id = id;
this.title = title;
this.contents = contents;
}
// ๊ธฐ๋ฅ
public static BoardResponseDto toDo(Board board) {
return new BoardResponseDto(board.getId(), board.getTitle(), board.getContents());
}
}
BoardController
@RestController
@RequestMapping("/boards")
@RequiredArgsConstructor
public class BoardController {
// ์์ฑ
private final BoardService boardService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@GetMapping
public ResponseEntity<List<BoardResponseDto>> findAll() {
List<BoardResponseDto> boardResponseDtoList = boardService.findAll();
return new ResponseEntity<>(boardResponseDtoList, HttpStatus.OK);
}
}
BoardService
@Service
@RequiredArgsConstructor
public class BoardService {
// ์์ฑ
private final MemberRepository memberRepository;
private final BoardRepository boardRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public List<BoardResponseDto> findAll() {
return boardRepository.findAll()
.stream()
.map(BoardResponseDto::toDo)
.toList();
}
}
9. ํน์ ๊ฒ์๊ธ ์กฐํ ๊ธฐ๋ฅ
ํน์ Board ์กฐํ API ๊ตฌํ
- ์๊ตฌ์กฐ๊ฑด : ์๋ต์ ์์ฑ์ ๋์ด๋ฅผ ํฌํจํ๋ค.
BoardWithAgeResponseDto
@Getter
public class BoardWithAgeResponseDto {
// ์์ฑ
private final String title;
private final String contents;
private final Integer age;
// ์์ฑ์
public BoardWithAgeResponseDto(String title, String contents, Integer age) {
this.title = title;
this.contents = contents;
this.age = age;
}
// ๊ธฐ๋ฅ
}
BoardController
@RestController
@RequestMapping("/boards")
@RequiredArgsConstructor
public class BoardController {
// ์์ฑ
private final BoardService boardService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@GetMapping("/{id}")
public ResponseEntity<BoardWithAgeResponseDto> findById(@PathVariable Long id) {
BoardWithAgeResponseDto boardWithAgeResponseDto = boardService.findById(id);
return new ResponseEntity<>(boardWithAgeResponseDto, HttpStatus.OK);
}
}
BoardService
@Service
@RequiredArgsConstructor
public class BoardService {
// ์์ฑ
private final MemberRepository memberRepository;
private final BoardRepository boardRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public BoardWithAgeResponseDto findById(Long id) {
Board findBoard = boardRepository.findByIdOrElseThrow(id);
Member writer = findBoard.getMember();
return new BoardWithAgeResponseDto(findBoard.getTitle(), findBoard.getContents(), writer.getAge());
}
}
BoardRepository
public interface BoardRepository extends JpaRepository<Board, Long> {
default Board findByIdOrElseThrow(Long id) {
return findById(id).orElseThrow(() -> new ResponseStatusException(HttpStatus.NOT_FOUND, "Does not exist id = " + id));
}
}
10. ํน์ ๊ฒ์๊ธ ์ญ์ ๊ธฐ๋ฅ
๊ฒ์๊ธ ์ญ์ API ๊ตฌํ
BoardController
@RestController
@RequestMapping("/boards")
@RequiredArgsConstructor
public class BoardController {
// ์์ฑ
private final BoardService boardService;
// ์์ฑ์
// ๊ธฐ๋ฅ
@DeleteMapping("/{id}")
public ResponseEntity<Void> delete(@PathVariable Long id) {
boardService.delete(id);
return new ResponseEntity<>(HttpStatus.OK);
}
}
BoardService
@Service
@RequiredArgsConstructor
public class BoardService {
// ์์ฑ
private final MemberRepository memberRepository;
private final BoardRepository boardRepository;
// ์์ฑ์
// ๊ธฐ๋ฅ
public void delete(Long id) {
Board findBoard = boardRepository.findByIdOrElseThrow(id);
boardRepository.delete(findBoard);
}
}
์ค๋ ํ๋ฃจ ์ ๋ฆฌ โ๏ธ
์ค๋์ ์ค์ ์ ์๊ณ ๋ฆฌ์ฆ ๋ฌธ์ Java๋ SQL ํ๋ฌธ์ ์ฉ ํ๊ณ ๋ธ๋ก๊ทธ์ ์ ๋ฆฌํ๋ค. ์ค๋๋ง์ SQL ๋ฌธ์ ๋ฅผ ํ์๋๋ฐ ์๊ฐ๋ณด๋ค ์ฝ๊ฒ ํ๋ ธ๋ค! ์์ ๊น๋จน๊ธฐ ์ ์ ๋ค์ ๋ฌธ์ ๋ฅผ ํ๊ธฐ ์์ํด์ ๋คํ์ด๋ผ๋ ์๊ฐ์ด ๋ค์๋ค.
์๊ณ ๋ฆฌ์ฆ ๋ฌธ์ ๋ฅผ ๋๋ด๊ณ ๋์๋ ์ญ Spring ์๋ จ 3์ฃผ์ฐจ ์ค์ต ๊ฐ์๋ฅผ ๋ค์๋ค. ๊ฐ์ฒด๋ฅผ ๋ง๋ค๊ณ JPA๋ฅผ ์ค์ตํ๋ ์๊ฐ์ด์๋๋ฐ ํ์คํ JDBC๋ฅผ ์ฌ์ฉํ๋ ๊ฒ๋ณด๋ค ํธ๋ฆฌํ ๋ฐฉ๋ฒ์ด๋ผ๋ ์๊ฐ์ด ๋ค์๋ค. JPA๋ฅผ ์ฌ์ฉํ๋ฉด ์ฝ๋๊ฐ ์์ฒญ ๊ฐ๋จํด์ก๋ค!!!! ๊ทธ๋์ ํฅ๋ฏธ๋กญ๊ฒ ๊ฐ์๋ฅผ ๋ฐ๋ผํ๋ฉด์ CRUD ๊ธฐ๋ฅ์ ์์ฑํ ์ ์์๋ค.
๊ทผ๋ฐ ๋ญ๊ฐ ๊ฐ์๋ฅผ ๋ค๋ฃ๊ณ ๋๋๊น ์ดํดํ๊ธฐ๋ ์ ์ ๊ทธ๋ฅ ์ฝ๋๋ฅผ ๋ฐ๋ผ์น๋ฉด์ ๊ตฌํ๋ง ํ ๋๋์ด๋ผ์ ์๋ก ํ๋ก์ ํธ๋ฅผ ๋ง๋ค๊ณ ๊ธฐ์ต์ ์์กดํ๋ฉด์ ๋ค์ ์ง์ ์ฝ๋๋ฅผ ์์ฑํด๋ดค๋ค. ๋ฌผ๋ก ๋ค ๊ธฐ์ตํ์ง ๋ชปํด์ ๊ฐ์ ์๋ฃ๋ฅผ ์ฐธ๊ณ ํ๋ฉด์ ์ฝ๋๋ฅผ ์ดํดํ๊ณ ํ๋ฆ์ ์ดํดํ๋ฉด์ ํ๋๊น ํจ์ฌ ๋จธ๋ฆฌ์ ์ ๋ค์ด์ค๋ ๋๋์ด ๋ค์๋ค. ์๊ฐ์ ๋ง์ด ๊ฑธ๋ ธ์ง๋ง ์ง์ ์์ฑํ ์ฝ๋๊ฐ ๋ง์ผ๋๊น ๋ฟ๋ฏํ๋ค ๐
์ค๋์ ๊ฐ์ ์ค์ต ๋ฐ๋ผํ๊ณ ๋ณต์ตํ๋ค๊ณ ํ๋ฃจ๋ฅผ ๋ค ์ด ๊ฒ ๊ฐ๋ค. ๋ด์ผ๋ถํฐ๋ ์ง์ง ๊ณผ์ ๋ฅผ ์์ํด์ผ์ง..
๊ณผ์ ๋ฅผ ์ต๋ํ ๋นจ๋ฆฌ ์์ํด์ ์ด๋ ค์ด ๋ด์ฉ๋ค์ ์ง์ ๋ถ๋ชํ๋ฉด์ ํด๊ฒฐํ๊ณ ํ์ธต ๋ ์ฑ์ฅํ๋ฉด ์ข์ ๊ฒ ๊ฐ๋ค! ๋ด์ผ๋ถํฐ ๊ณผ์ ๋ฅผ ์์ํ๋ฉด ์ง์ด ์ญ์ญ ๋น ์ง๊ฒ ์ง๋ง ์ค๊ฐ์ค๊ฐ ์ ์ฌ์ด๊ฐ๋ฉด์ ์งํํ๋ฉด ๋ฌธ์ ์์ ๊ฒ ๊ฐ๋ค!
์ค๋ ํ๋ฃจ๋ ์ด์ฌํ ๊ณต๋ถํ๊ณ ๋ด์ผ๋ง ์ ๋ณด๋ด๋ฉด ๋ ์ฃผ๋ง์ด๋๊น ์ด์ฌํ ๊ณต๋ถํ์!!! ํ์ดํ !!
๋ด์ผ ๊ณํ โฐ
- ์๊ณ ๋ฆฌ์ฆ ๋ฌธ์ (Java, SQL) 1๋ฌธ์ ํ์ด & ๋ธ๋ก๊ทธ ์ ๋ฆฌ
- ๊ณผ์ ์์ํด๋ณด๊ธฐ(?)
- TIL ๋ธ๋ก๊ทธ ์์ฑ
+ ์ถ๊ฐ๋ก ๊ณํ์ด ๋ ์๊ธธ ์๋ ์์ด๋ค~_~
'Today I Learned(TIL) > ์คํ๋ฅดํ ๋ด์ผ๋ฐฐ์์บ ํ' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
์ฃผํน๊ธฐ ์ ๋ฌธ/์๋ จ_Day 16 (1) | 2024.12.14 |
---|---|
์ฃผํน๊ธฐ ์ ๋ฌธ/์๋ จ_Day 15 (1) | 2024.12.13 |
์ฃผํน๊ธฐ ์ ๋ฌธ/์๋ จ_Day 13 (0) | 2024.12.11 |
์ฃผํน๊ธฐ ์ ๋ฌธ/์๋ จ_Day 12 (0) | 2024.12.10 |
์ฃผํน๊ธฐ ์ ๋ฌธ/์๋ จ_Day 11 (2) | 2024.12.09 |